Connecting a micro SD card reader to an Arduino Nano RP2040 Connect
I get an error message from the code [ Initializing SD card...initialization failed! ] which means that the SD card reader has failed to be recognized by the chip select pin chosen.
I have used pin 4, 10, 28, and 7. No difference.
Am I doing something basically wrong ?
Here is the code, which Nill has demonstrated.
#########################################
/*
SD card read/write
This example shows how to read and write data to and from an SD card file
The circuit:
* SD card attached to SPI bus as follows:
** MOSI - pin 11
** MISO - pin 12
** CLK - pin 13
** CS - pin 4 (for MKRZero SD: SDCARD_SS_PIN )
28 ( for RP2040 Nano Connect: QSPI_CSn )
created Nov 2010
by David A. Mellis
modified 9 Apr 2012
by Tom Igoe
modified and extended 20 July 2023
by Chris. J. Muller
This example code is in the public domain.
*/
#include <SPI.h>
#include <SD.h>
#define CS_PIN 4
File myFile;
String file= "test.txt";
void init_serial()
{
// Open serial communications and wait for port to open:
Serial.begin(9600);
while(!Serial)
{
; // wait for serial port to connect. Needed for native USB port only
}
}
void init_sd_card()
{
Serial.print("Initializing SD card...");
if(!SD.begin(CS_PIN))
{
Serial.println("initialization failed!");
while(1);
}
Serial.println("initialization done.");
}
void open_sd_card_to_write(String filename)
{
// open the file. note that only one file can be open at a time,
// so you have to close this one before opening another.
myFile = SD.open(filename, FILE_WRITE);
}
void error_opening_file(String filename)
{
// if the file didn't open, print an error:
Serial.print("error opening [ ");
Serial.print(filename);
Serial.println(" ]");
}
void sd_card_write_test(String filename)
{
// if the file opened okay, write to it:
if(myFile)
{
Serial.print("Writing to test.txt...");
myFile.println("testing 1, 2, 3.");
// close the file:
myFile.close();
Serial.println("done.");
}
else error_opening_file(filename);
}
void sd_card_read_test(String filename)
{
// re-open the file for reading:
myFile = SD.open(filename);
if(myFile)
{
Serial.print(filename);
Serial.println(":");
// read from the file until there's nothing else in it:
while(myFile.available())
{
Serial.write(myFile.read());
}
// close the file:
myFile.close();
}
else error_opening_file(filename);
}
void sd_card_test(String filename)
{
sd_card_write_test(filename);
sd_card_read_test(filename);
}
void setup()
{
init_serial(); // OKAY.
init_sd_card(); // RAISES A FAULT AND PROCEEDS NO FURTHER.
open_sd_card_to_write(file);
sd_card_test(file);
}
void loop()
{
// nothing happens after setup
}
#########################################
Please can anyone shed any light on the problem, or help me resolve the issue ?
Regards cjm3115
C J MULLER
@cjm3115
Is your SD card formatted ?
Anything seems possible when you don't know what you're talking about.
@cjm3115
Is your SD card formatted ?
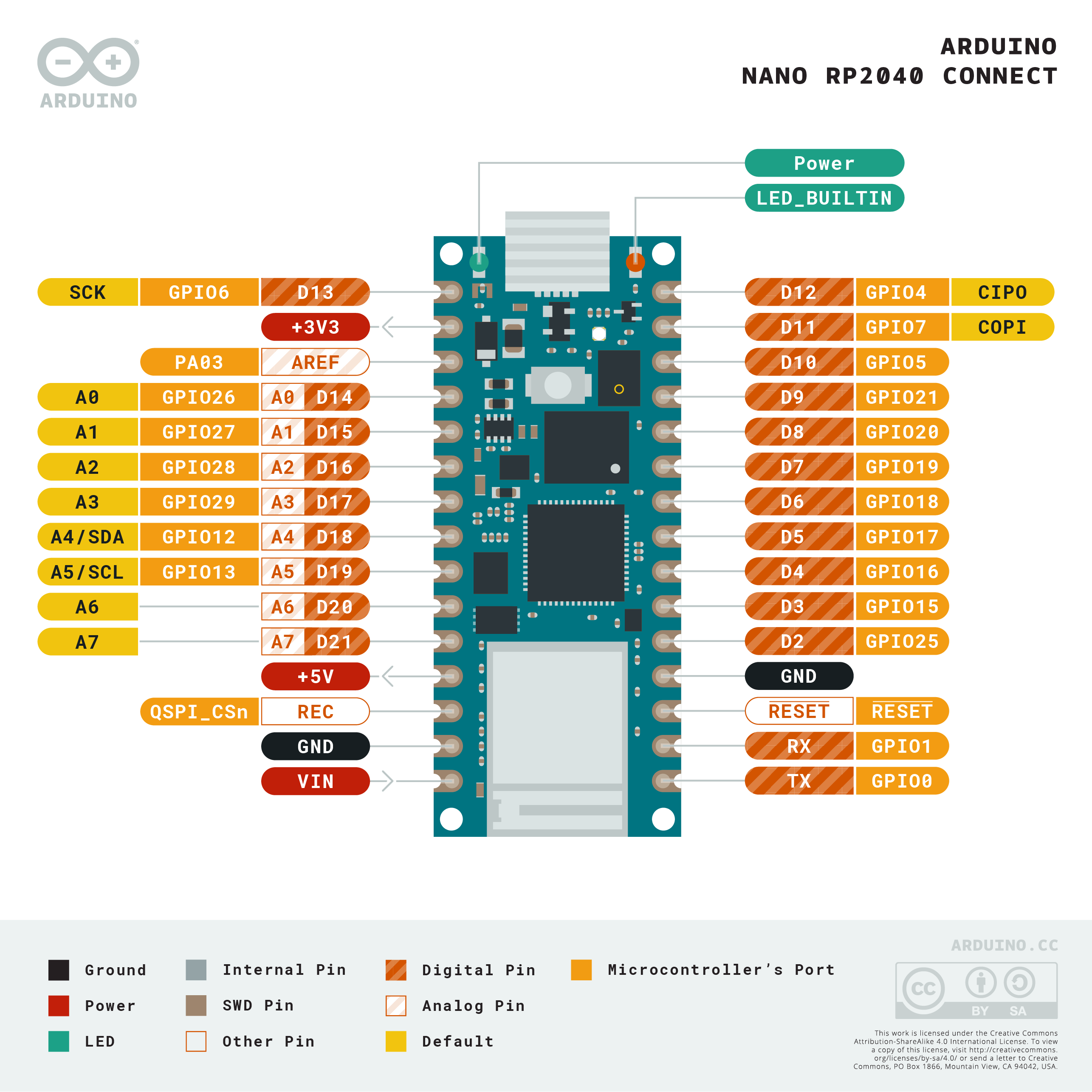
C J MULLER
I don't have a Connect to test with, have you tried formatting as FAT16 ?
Anything seems possible when you don't know what you're talking about.
@will No, I haven't tried FAT16 yet. I don't have the possibility of doing that anyway. Unless you can suggest how I can format the SD cards FAT16.
C J MULLER
Unless you can suggest how I can format the SD cards FAT16.
On your Mac, insert the SD card into a suitable device. Start up Disk Utility, select the drive, click erase, Click the dropdown for Format and select MS-DOS (FAT) and then click Erase to erase and reformat the SD card.
Anything seems possible when you don't know what you're talking about.
@cjm3115 It might help to copy/paste the actual error message.
First computer 1959. Retired from my own computer company 2004.
Hardware - Expert in 1401, and 360, fairly knowledge in PC plus numerous MPU's and MCU's
Major Languages - Machine language, 360 Macro Assembler, Intel Assembler, PL/I and PL1, Pascal, Basic, C plus numerous job control and scripting languages.
Sure you can learn to be a programmer, it will take the same amount of time for me to learn to be a Doctor.
I guess that 'const int' is preferred, because that is the type being passed to the function SD.start();. I will try this tomorrow and thank you for your help and advice. On the off chance that this doesn't work, have you any other suggestions ?
Regards cjm3115.
C J MULLER
The process actually fails here, and I am now using
const int= 10;
###
Fails here :-
Serial.print("Initializing SD card...");
if(!SD.begin(10))
{
Serial.println("initialization failed!");
while(1);
}
Serial.println("initialization done.");
###
SD.begin(10) never completes, so the 'if condition' is then true with 'while(1);' preventing the code from progressing any further.
The issue arises in 'SD.begin(cs_pin)' , where I have set cs_pin as follows :
const int cs_pin= 10;
Can you help me ?
Do I have to look inside the 'SD' library code ?
C J MULLER
I succeeded in formatting the SD card to FAT16, but this did not resolve the issue, this remains with 'SD.begin(10)', please remember that I am using an Arduino Nano RP2040- Connect and not another Arduino.
I am tending to think that the code issue has arisen, because I am using an Arduino Nano RP2040- Connect and something in the 'SD' library is not compatible to allow 'SD.begin(10)' to complete successfully.
What do you think ?
C J MULLER
I looked inside the SD library and related code, but I couldn't find any obvious code which could apply only to certain Arduinos pertaining to the SPI chip select pin.
I would still appreciate anyone's help with this problem, so that I can resolve the issue causing 'SD.start' not to complete successfully.
Happy Coding.
C J MULLER
@cjm3115 Sorry to be the messenger, but you need to address your comments by using the Reply link or adding @handle prior to the message.
First question is which SD library are you using?
I installed the Arduino SD library and loaded the datalogger sample. It's setup is as follows.
void setup() { // Open serial communications and wait for port to open: Serial.begin(115200); Serial.print("Initializing SD card..."); // Ensure the SPI pinout the SD card is connected to is configured properly SPI.setRX(_MISO); SPI.setTX(_MOSI); SPI.setSCK(_SCK); // see if the card is present and can be initialized: if (!SD.begin(_CS)) { Serial.println("Card failed, or not present"); // don't do anything more: return; } Serial.println("card initialized."); }
The pins are
const int _MISO = 4; const int _MOSI = 7; const int _CS = 5; const int _SCK = 6;
First computer 1959. Retired from my own computer company 2004.
Hardware - Expert in 1401, and 360, fairly knowledge in PC plus numerous MPU's and MCU's
Major Languages - Machine language, 360 Macro Assembler, Intel Assembler, PL/I and PL1, Pascal, Basic, C plus numerous job control and scripting languages.
Sure you can learn to be a programmer, it will take the same amount of time for me to learn to be a Doctor.
@cjm3115 Which SD code did you look at (please provide the full path of the library)
There is also a compile error in the version of the code you posted first. The error message includes the fix.
First computer 1959. Retired from my own computer company 2004.
Hardware - Expert in 1401, and 360, fairly knowledge in PC plus numerous MPU's and MCU's
Major Languages - Machine language, 360 Macro Assembler, Intel Assembler, PL/I and PL1, Pascal, Basic, C plus numerous job control and scripting languages.
Sure you can learn to be a programmer, it will take the same amount of time for me to learn to be a Doctor.